As a Network Engineer, I've come to realize that Python is no longer something we can afford to ignore. The world of Network Engineering is rapidly evolving, and at the heart of this transformation is Network Automation or NetDevOps. Python has become a central player in this shift, offering powerful and flexible solutions for Automating Network tasks.
Now, I know what some of you might be thinking, "I already use Ansible for Network Automation, so why should I bother learning Python?" That's a fair question. Ansible is a fantastic tool and has its place in our toolbox, but Python opens up a whole new world of possibilities.
And for those of you who are just starting out with Network Automation, it can be pretty overwhelming to decide which tool to pick up first. Is it Ansible, Python, or some other tool? It's a dilemma, I get it.
In this blog post, I'm going to dive into why learning Python is such a crucial step for us Network Engineers. I'll be sharing some real-world use cases and examples to illustrate just how valuable Python can be in our field.
Ansible vs Python
Ansible is a popular Automation tool that uses a declarative language called YAML to define configuration management tasks. A declarative language is used to tell a system what you want it to do, without having to give it specific instructions on how to do it. In simpler terms, you simply state what you want the system to accomplish, and the computer figures out the details of how to do it.
Python is a general-purpose programming language that is also used for Network Automation. Python provides a wide range of libraries (Netmiko, Nornir, Napalm) and frameworks that can be used to automate tasks, making it a versatile tool for Network Automation. Python provides more flexibility and control over Ansible. A programming language is like giving someone a set of instructions to follow. You tell them exactly what to do and how to do it, step-by-step.
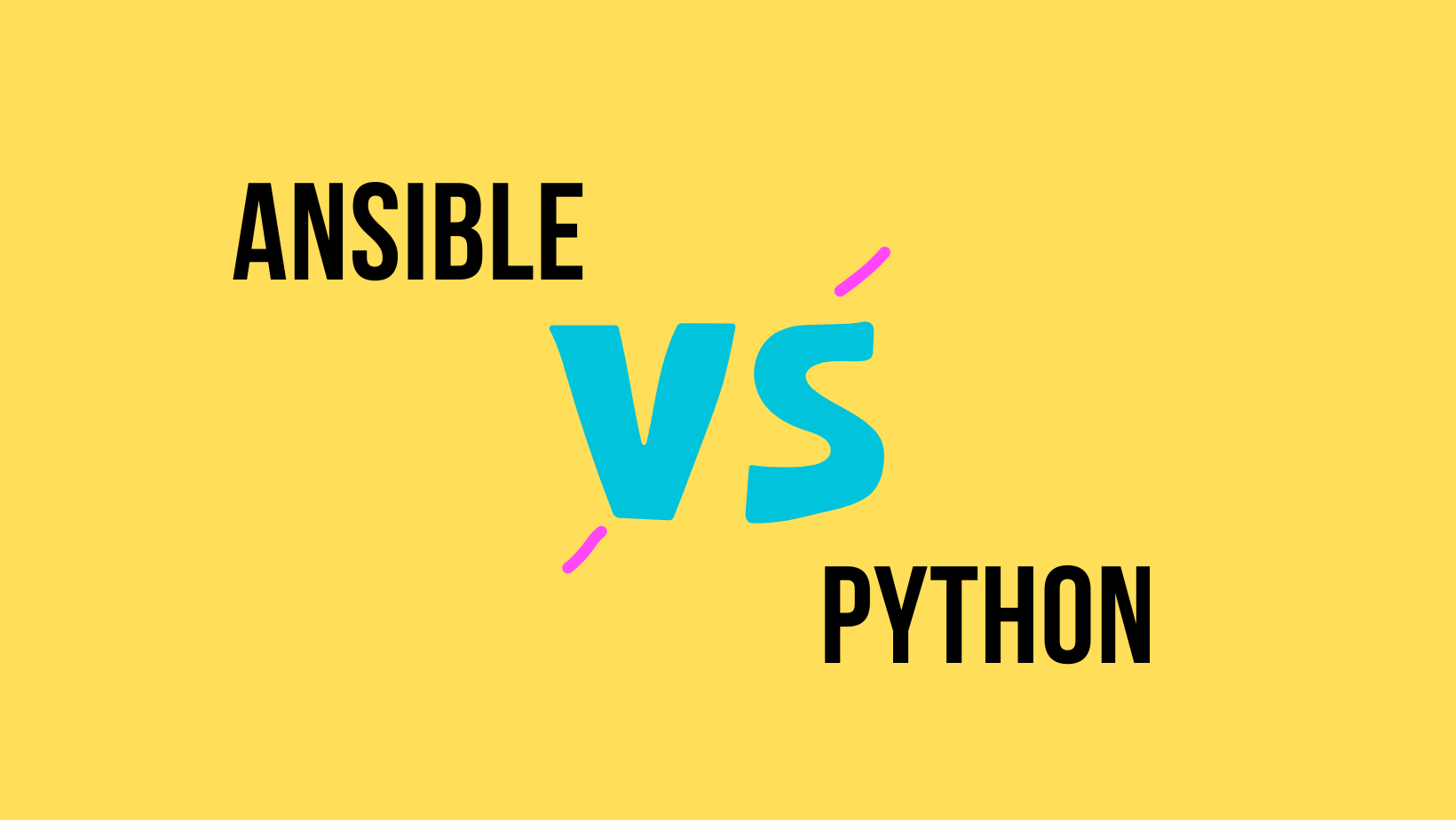
Ansible Limitations
Though Ansible is undeniably powerful and user-friendly, there comes a point where you might hit a wall with its capabilities. Consider the diverse range of automation options provided by different networking vendors. For instance, Cisco offers interfaces like Restconf or Netconf, and Palo Alto has options like REST-API and Python SDK. These varied interfaces can sometimes pose challenges for Ansible's more standardized approach.
This is where Python truly shines. With Python, the possibilities are practically limitless. You have the freedom to interact with a wide array of vendor-specific APIs and tools, giving you a level of flexibility and control that Ansible, in some scenarios, may not be able to match. Python acts as a universal key, unlocking doors that might remain closed when using a tool like Ansible.
Python Simple Use Cases
In my experience as a Network Engineer, I've found that the best way to get comfortable with Python is to use it daily in your work environment. It's a hands-on approach that makes learning much more effective.
If you're a Network Engineer, chances are there are still numerous tasks you're doing manually. My advice? Start automating them with Python. It's easier than you might think, and the benefits are huge.
Configuration Backup
Let's take a common scenario, backing up configurations across multiple devices. Instead of manually copying and saving configurations, you can write a Python script to do it for you. Here’s a simple script that I’ve used.
from netmiko import ConnectHandler
import getpass
passwd = getpass.getpass('Please enter the password: ')
file_dir = '/Users/suresh/Documents/config-backup/backups'
switch_list = ['192.168.10.10', 'core-switch-01', 'test_switch']
device_list = []
for ip in switch_list:
device = {
"device_type": "cisco_ios",
"host": ip,
"username": "suresh",
"password": passwd,
"secret": passwd # Enable password
}
device_list.append(device)
for device in device_list:
host_name = device['host']
connection = ConnectHandler(**device)
show_run = connection.send_command('show run')
with open(f"{file_dir}/show_run_{host_name}.txt", 'w') as f:
f.write(show_run)
connection.disconnect()
This script connects to the devices listed and backs up their configurations. It's straightforward, but it shows the power of Python in NetworkAutomation. With just a few lines of code, you can automate a task that would otherwise be tedious and time-consuming.
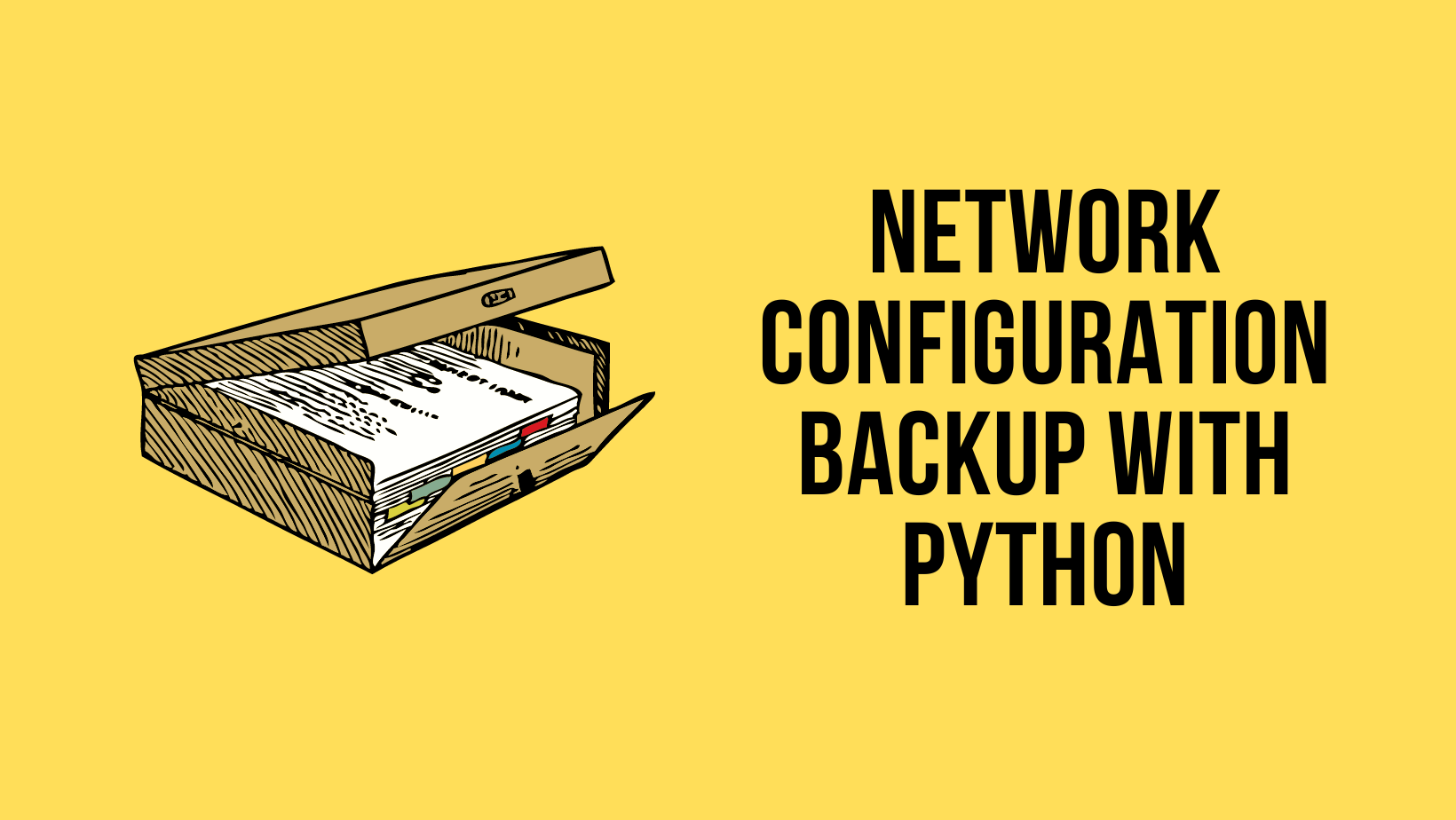
VLAN Configuration
Have you ever found yourself in a situation where you needed to standardize the configuration of VLAN names across multiple switches? Recently, a friend of mine faced a similar challenge and reached out to me for help. I decided to use my Python skills to come up with a solution. Here is a Python script that would standardize the VLAN names across 100s of switches.
- I have a list of VLANs to work with that have the VLAN ID and the correct Name.
- If a particular VLAN doesn't exist on a switch, don't create it.
- If a particular VLAN does exist on a switch but has a wrong name, the script should change the name to the correct one.
- The script should never delete or create new VLANs.
from netmiko import ConnectHandler
switch_list = ['10.10.20.18', '10.10.20.19', '10.10.20.20']
device_inventroy = []
vlans = {
'10': 'MGMT',
'20': 'DATA',
'30': 'active_directory',
'31': 'web_servers',
'32': 'admin',
'33': 'network'
}
for ip in switch_list:
device = {
"device_type": "cisco_ios",
"host": ip,
"username": "cisco",
"password": 'cisco123',
"secret": 'cisco123' # Enable password
}
device_inventroy.append(device)
for switch in device_inventroy:
connection = ConnectHandler(**switch)
output = connection.send_command('show vlan', use_textfsm=True)
connection.enable() # Enable method
connection.config_mode() # Global config mode
current_vlans_dict = {}
for vlan in output:
current_vlans_dict[vlan['vlan_id']] = vlan['name']
for k,v in vlans.items():
if k in current_vlans_dict and v != current_vlans_dict[k]:
commands = [f"vlan {k}", f"name {v}"]
config_output = connection.send_config_set(commands)
print(config_output)
connection.disconnect()
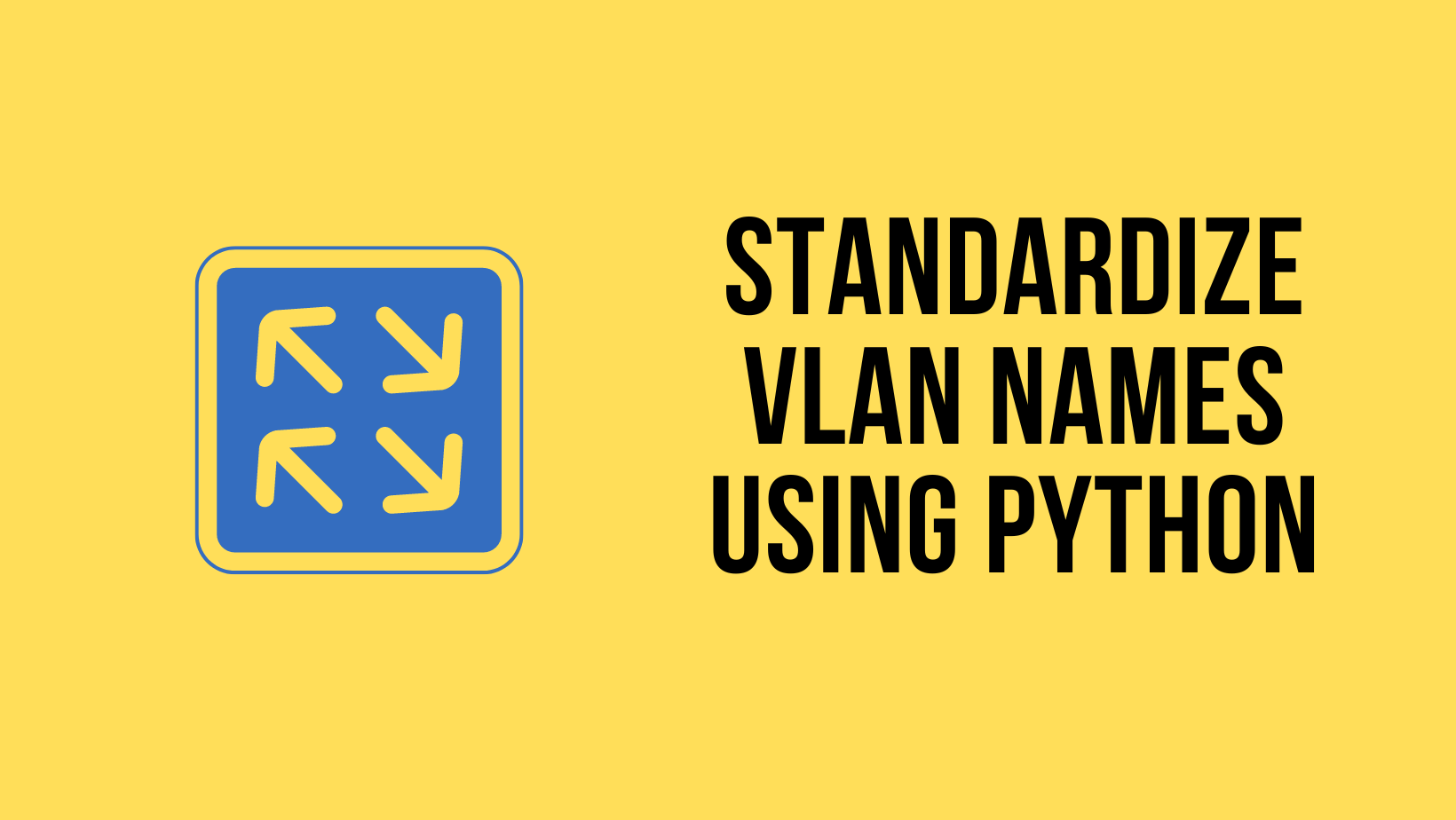
Some Advanced(ish) Use Cases
Now, let’s delve into some more advanced (or let's say, advanced-ish) use cases where Python really proves its worth in NetworkAutomation.
Cisco/Palo Alto
I'm not going to dive into specific examples here, but rather give you an idea of the scenarios where Python can be used. Take my work with Palo Alto firewalls, for instance. Palo Alto offers several options for automation, like XML API, REST API, and a Python SDK. While you can use XML or REST API without Python, pairing them with Python significantly simplifies the process.
Using Python in conjunction with Palo Alto's Python SDK, I can configure firewalls with much more flexibility and precision. It's a powerful combination that allows for more complex, customized configurations than what you might achieve with standard tools alone.
Similarly, when dealing with Cisco devices, you've got options like Netconf and Restconf. These are protocols designed for network configuration, and Python serves as the perfect tool to interact with these protocols programmatically. Here are some of the examples. Here are some of my blog posts on Palo Alto Automation.
AWS
Let's talk about cloud environments, specifically AWS. AWS's Python SDK, known as Boto3, is a tool I use frequently and it's a perfect example of Python's versatility.
Imagine a scenario where you need to perform actions in AWS based on certain events or triggers. This could be anything from terminating an instance to creating a network interface. Here's where Python, combined with AWS Lambda, becomes incredibly useful.
With AWS Lambda, you can run Python scripts in response to specific AWS events without needing to provision or manage servers manually. For example, I might write a Python script that uses Boto3 to monitor for specific conditions or events in my AWS environment. Once these conditions are met, the script can automatically execute tasks like modifying network configurations and launching or stopping instances.
Closing Up
Just to sum it up, learn Python and try to use it in your day-to-day tasks. Here are some of the blog posts I've written on how to use Python for Network Automation. If you are have any comments or feedbacks, please let me know in the comments.