A few years ago, I used a simple application called 'TypeItIn'. It kept a small GUI window open with some buttons and labels. You could configure each label with your own text. If you wanted to type one of these texts into a window, all you needed to do was click on the label, and it would start typing the text into whatever window you opened. It was such a time-saver, especially if you had multiple texts that you often used.
Fast forward a few years, I really needed such a tool and then realized I knew a bit of Python, so I should be able to create the same functionality using Python. So, in this blog post, let's go through how you can create a simple GUI application with just a few lines of code.
What Is Tkinter?
Tkinter is the standard GUI toolkit for Python, providing a fast and easy way to create simple GUI applications. It is built into Python, so there’s no need to install anything separately if you already have Python.
Tkinter is widely used due to its simplicity and the vast availability of widgets like buttons, menus, and text fields, which help in building custom applications quickly.
Here Is the Script
In this script, I’ve set up a simple Python application using Tkinter to create a user interface that allows you to quickly copy predefined texts to your clipboard.
.
├── content.yaml
└── main.py
1 directory, 2 files
The process starts by reading data from a YAML file named content.yaml
This file contains a list of items, each represented as a dictionary with two keys called label and text. The label is what you’ll see on the button in the GUI, and the text is what gets copied to your clipboard when you click the button.
items:
- label: "Blog Intro"
text: "Hi all welcome back to my blog."
- label: "Another label"
text: "This is a test label."
- label: "Quick Tip"
text: "Remember to check back for weekly updates!"
- label: "Contact Info"
text: "Email us at contact@example.com for more information."
- label: "Disclaimer"
text: "All opinions expressed here are my own and not those of my employer."
I use the PyYAML library to read this file. A function called yaml_read
opens the YAML file and parses its contents into a Python dictionary.
import tkinter as tk
import yaml
def yaml_read(yaml_file):
with open(yaml_file, 'r') as f:
data = yaml.load(f, Loader=yaml.SafeLoader)
return data
data = yaml_read('content.yaml')
def copy_to_clipboard(text):
root.clipboard_clear()
root.clipboard_append(text)
def create_button(item):
return tk.Button(root, text=item["label"],
command=lambda: copy_to_clipboard(item["text"]))
root = tk.Tk()
root.title("Copy to Clipboard")
root.geometry("300x150")
# Using the updated items data structure
items = data['items']
for item in items:
btn = create_button(item)
btn.pack()
root.mainloop()
The Tkinter library is used to build the window for the application. It’s set to a manageable size and titled 'Copy to Clipboard'. For each item in the list from the YAML file, a button is created. These buttons are generated using a function called create_button
, which takes the item’s label and text. When a button is pressed, it triggers another function called copy_to_clipboard
, which clears the current clipboard contents and copies the button’s associated text.
The btn.pack()
method in Tkinter is used to manage the placement of widgets within the window. The pack()
method is part of the geometry management system in Tkinter. By calling btn.pack()
, the button widget btn
is automatically sized and positioned according to its contents and optionally, additional parameters that control the layout.
The root.mainloop()
is a crucial part of any Tkinter application. This method call starts the application's event loop, which waits for events such as keystrokes or mouse clicks and processes them as long as the window is open.
Each button is displayed in the main window, and clicking on any of them will instantly copy the corresponding text, making it ready to paste wherever you need it.
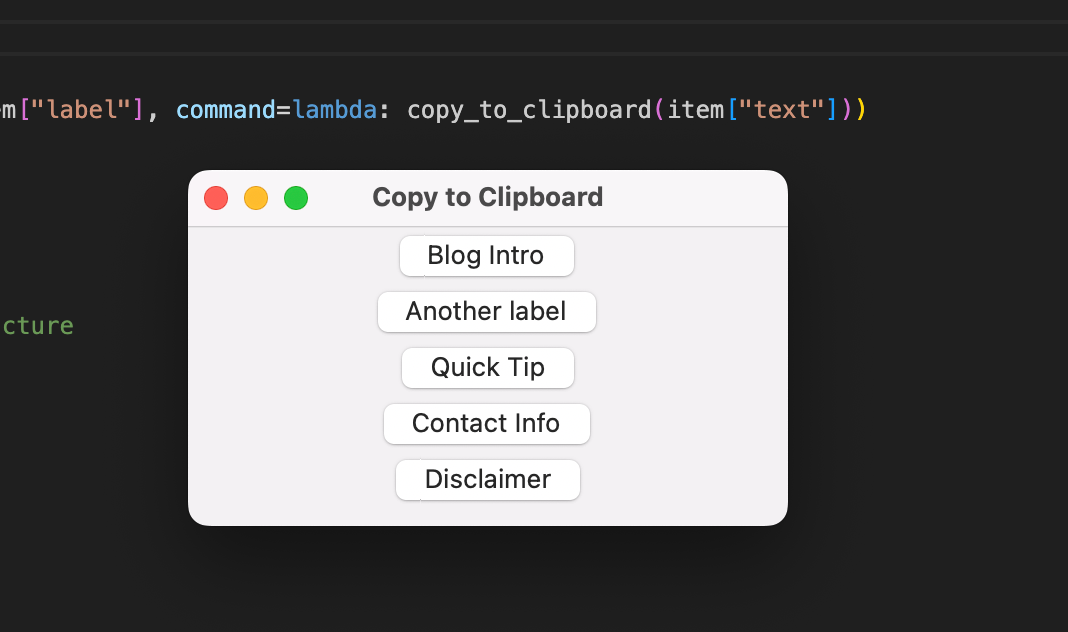
Keeping the Window at the Top
If you want to keep the application window always on top of other windows, you can use the root.attributes('-topmost', True)
command in your Tkinter script.
Just add this line to your script after configuring the window’s size and title, and your application will stay in the forefront on your desktop.
root.attributes('-topmost', True)
Closing Up
I'm planning to learn more about Tkinter and see if I can make improvements to this tool. Please make sure to subscribe so that when I publish new articles, they will come right to your inbox.