Hi all, welcome to the journey of Python for Network Engineers. I'm thrilled to guide you through this exciting path. Let me start off by saying Python is incredibly approachable and straightforward to learn. With just a few weeks of dedication, you can understand the basics.
Understanding the basics well is the most important part of this journey, everything you build on top will be stronger and more reliable. This solid foundation is key to your long-term success.
My aim here is to keep everything very simple and straightforward. I believe in cutting through the complexity and making learning accessible and enjoyable. We'll cover the basics of Python in a way that directly applies to network engineering, showing you how this powerful language can make your life easier and your work more efficient.
All of my study guides/courses come with one monthly session (one hour) (for paid-members) where we can dive deeper into any topics of interest or tackle any questions or concerns you might have. This is a great opportunity for interactive learning and getting personalized advice.
Our End Goal
Our end goal here is clear, by the end of this course, you will be familiar with Python syntax, comfortable creating your own Python code, and able to configure/manage network devices as well as automate smaller manual tasks.
I aim to teach you the skills needed to not just understand Python from a theoretical standpoint but to apply it practically in your day-to-day network tasks. Whether it's automating repetitive tasks, managing device configurations, or simply making your workflow more efficient, this course is designed to get you there.
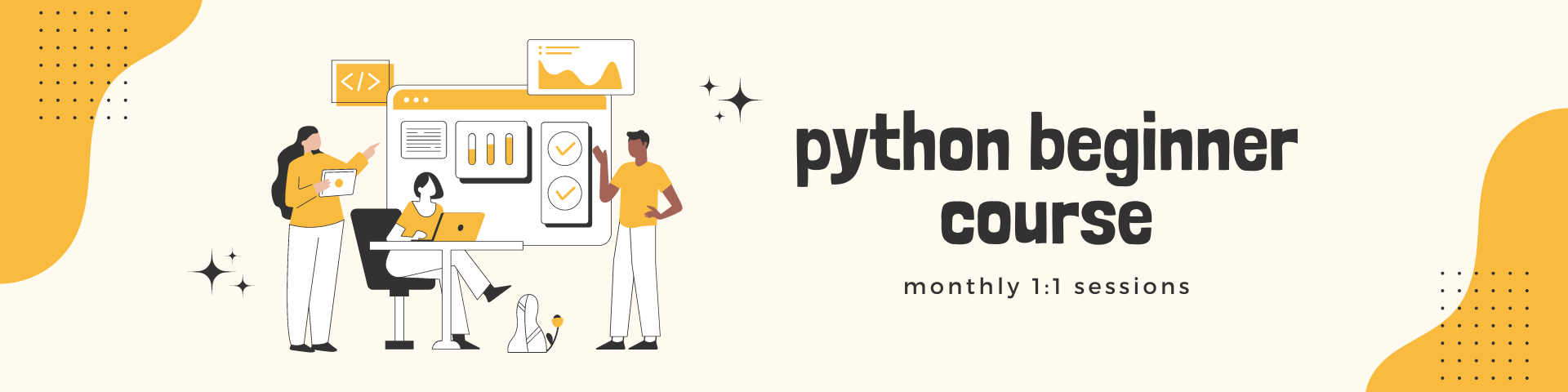
What We Will Learn?
In this course, we'll cover everything you need to get started with Python. We'll begin by showing you how to install Python on your machine. Then, we'll dive into running your first 'Hello World!' program—a traditional way to start your programming journey.
From there, we'll explore the basics of programming in Python, including variables, data types, operators, loops, and functions. These are the building blocks of any Python program, and understanding them is crucial for your success.
We'll also introduce you to Netmiko, a powerful Python module specifically designed for managing network devices. By the end of this course, you'll not only be comfortable with Python syntax but also capable of using Netmiko to automate tasks and manage network configurations.
- Installing Python on your machine
- Running your first "Hello World!" program
- Variables - Understanding and using variables
- Data Types:
- Strings
- Integers
- Floats
- Lists
- Tuples
- Dictionaries
- Booleans
- Expressions and Operators:
- Arithmetic operators
- Comparison operators
- Logical operators
- Loops:
- For loops
- While loops
- Functions - Defining and calling functions
- Importing Modules
- PIP
- Netmiko - Using Netmiko for network device management
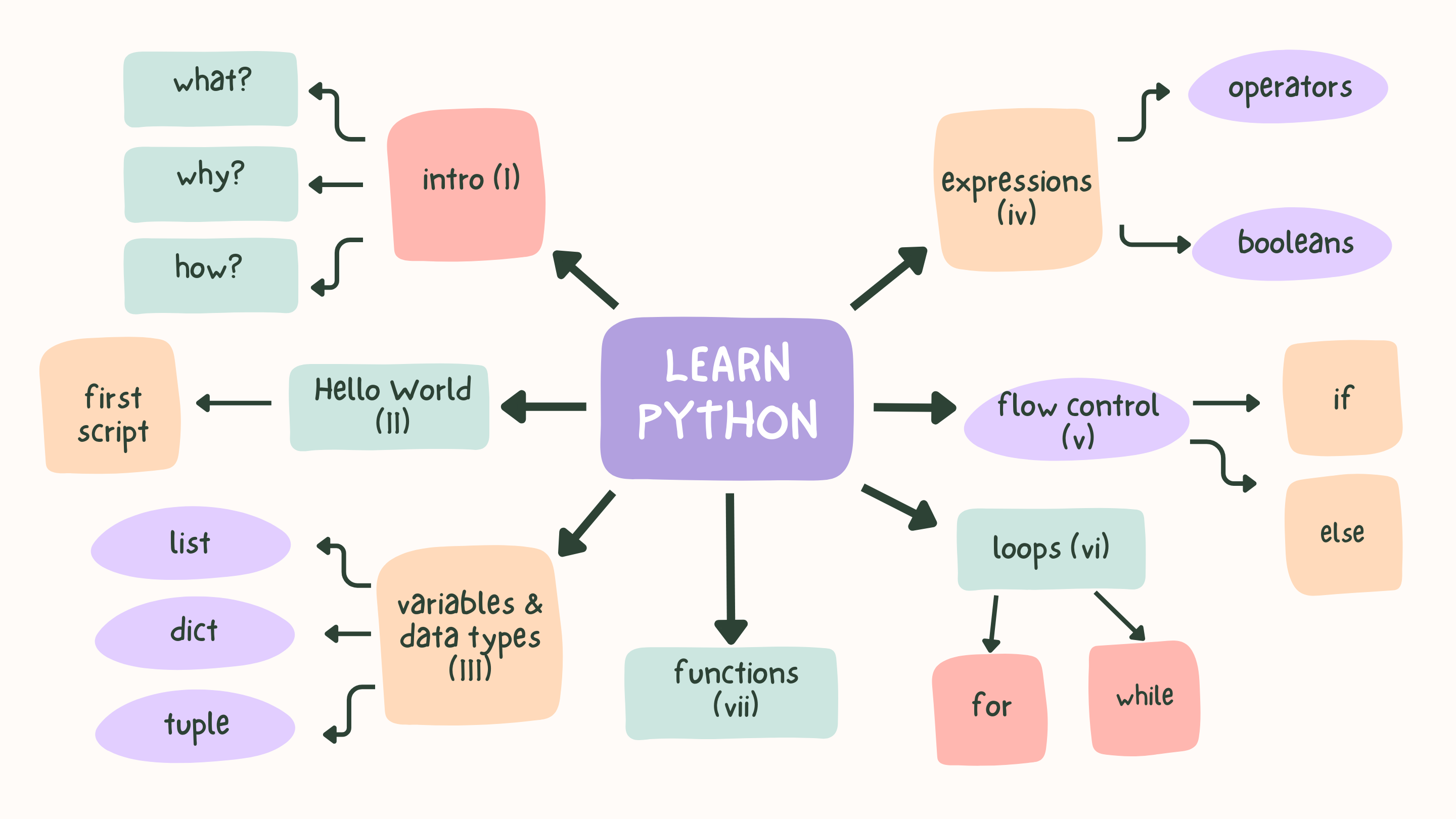
We Will Also Touch On
- Practical Examples and Case Studies - We'll dive into real-world examples, demonstrating how Python can be leveraged to solve common network issues and automate manual tasks. These examples will help you see the practical value of what you're learning.
- Introduction to APIs - You'll learn how to use Python to interact with network device APIs, such as REST APIs.
- Version Control with Git - An introduction to the basics of version control will help you manage and track changes in your code.
- Future Learning Path - Finally, we'll suggest pathways for further learning.
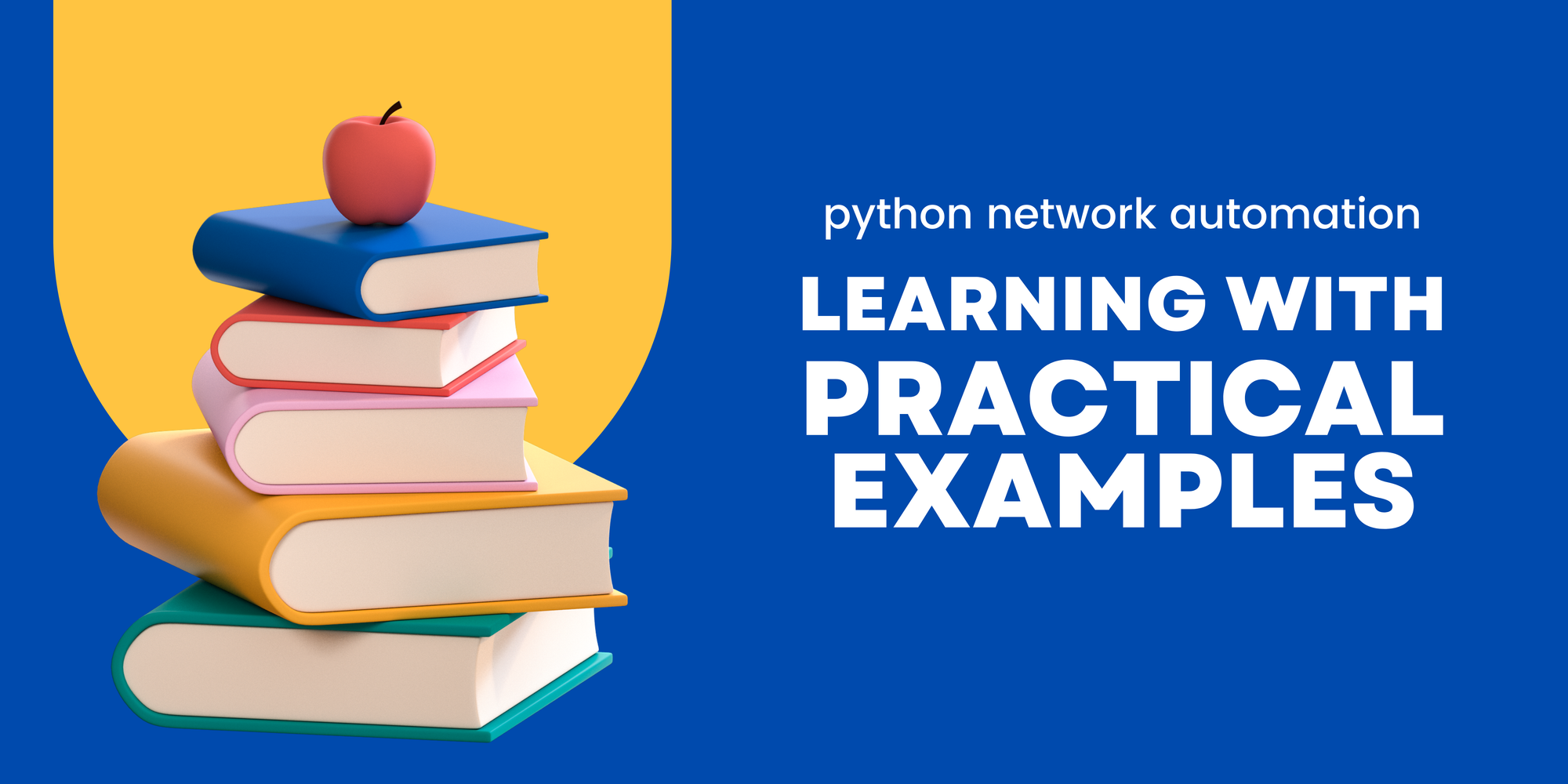
Why Python?
Before diving into learning Python, it's beneficial to understand why it has become such a critical skill, especially in the IT and networking field.
For Network Engineers, the move toward Network Automation underscores the value of Python. The ability to automate repetitive tasks, configure devices programmatically, and manage network operations more efficiently are just a few reasons why Python is becoming indispensable. With a rich ecosystem of modules like Netmiko, Nornir, Scrapli, and Napalm, Python provides the tools necessary for robust network automation solutions. Furthermore, many vendors support Python through their SDKs, such as panos-python
for Palo Alto Networks and PyEZ
for Juniper's Junos, making it easier to integrate Python into various network environments.
This wide-ranging support and the push towards automation make Python an ideal choice for beginners looking to make an impact in the IT and networking world. Learning Python not only opens the door to automating mundane tasks but also paves the way for innovative solutions to complex network challenges.
Learning Roadmap
Learning Python varies from person to person, so there's no strict path to follow. My advice? Take your time and make sure you really understand each part before moving on. Don't skip over things you find tough, as it'll only make future learning harder. Practice is key—write code, try out examples, and apply what you learn to real tasks, especially those related to your work. This hands-on experience is invaluable. And remember, the Python community is huge and supportive, so don't shy away from asking for help or sharing what you've learned.
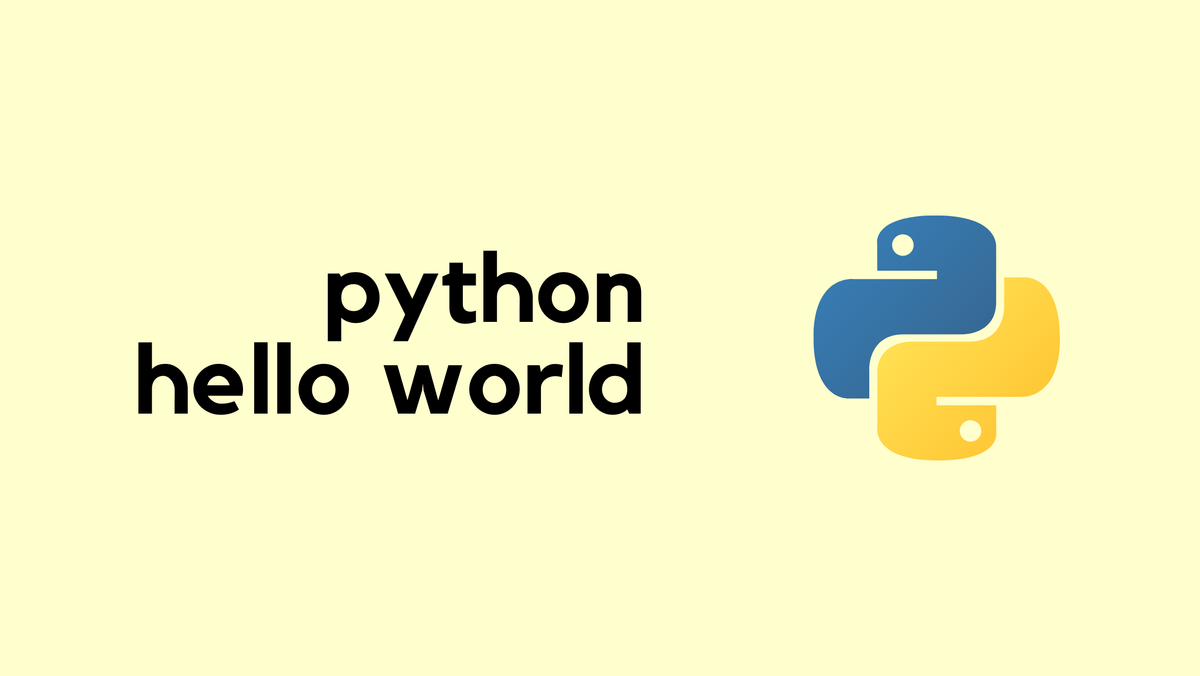