Once you’re comfortable with basic Python and can write simple scripts, the next step is learning about object-oriented programming and classes. There are plenty of resources out there explaining what classes in Python are, but I like to approach it from a different angle, asking “Why do we need this?” Because of that, I decided to write a blog post explaining not just how classes work, but also why they’re useful and how to apply them.
As always, if you find this post helpful, press the ‘clap’ button. It means a lot to me and helps me know you enjoy this type of content.
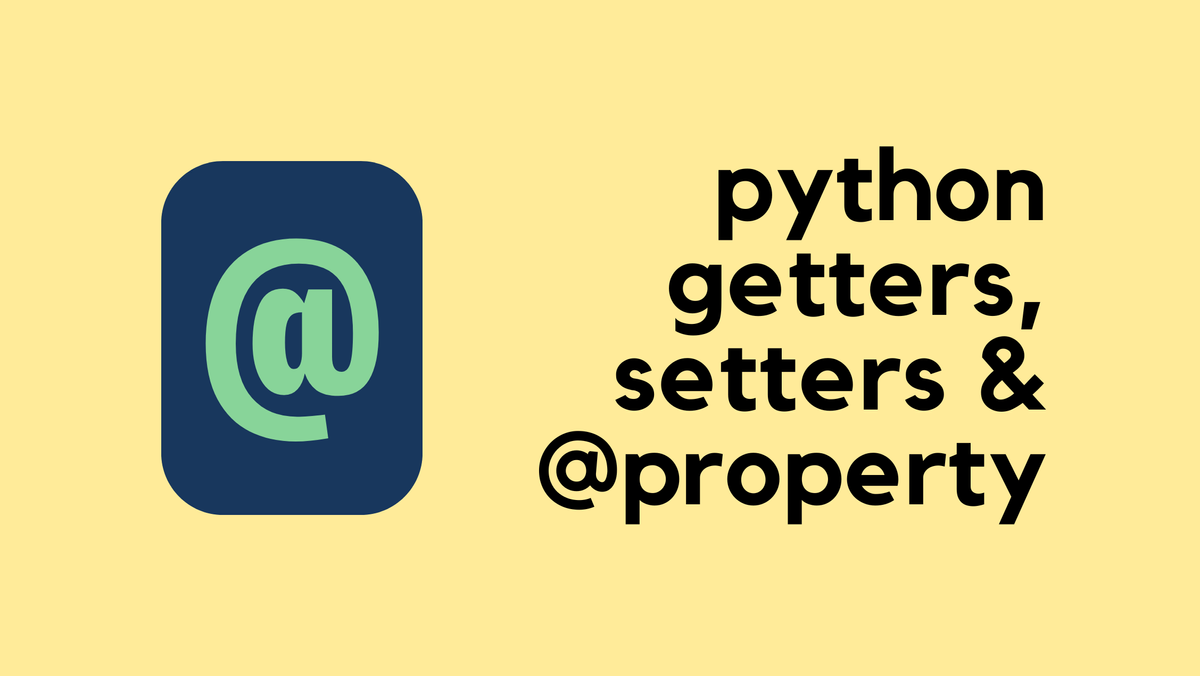
Overview
Think of a class as a way to group related data and behaviour together. If you’ve been writing Python scripts using functions, you might wonder, “Why do I need classes when functions already do the job?”
Let’s say you’re writing a program to manage a list of employees. Using functions, you might have one function to add an employee, another to update their salary, and another to print their details. But as your program grows, keeping track of all these separate functions and the data they operate on can become messy.
A class lets you bundle everything related to an employee into one place. You can store their name, salary, and other details inside the class, along with methods (similar to functions) that let you update or retrieve that information. This makes your code easier to manage and reuse.
So, instead of passing around multiple variables to functions, you can create an employee object that keeps everything in one place. It’s like having a template that ensures every employee has the same set of details and actions.
Now, before we move forward, I want to clarify something - I'm not saying you have to use classes all the time. It's not a fair comparison to say that functions are bad and classes are better. Functions are great for many use cases, and in some scenarios, using a class might be unnecessary. The reason I introduced functions first is simply to show how managing related data and behaviour separately can get messy as a program grows. Classes provide a structured way to bundle data and actions together, making things easier to manage in larger projects.
For small scripts or quick tasks, functions might be the simplest and best option. But as your code grows and you find yourself passing around the same data between multiple functions, that’s when a class might be a good fit. The key is knowing when to use which approach based on the problem you’re solving.
Managing Employees with Functions
Let's say we have a few employees, and we want to manage their details, such as their name and salary. Just using functions, can be a little difficult.
When using functions, we need to store employee details in a list or dictionary, and every function that modifies an employee's details has to search for the right employee. As the program grows, this can get messy, and maintaining consistency becomes harder. Here’s an example of how we might manage employees using functions.
employees = []
def add_employee(name, salary):
employees.append({'name': name, 'salary': salary})
def update_salary(name, new_salary):
for emp in employees:
if emp['name'] == name:
emp['salary'] = new_salary
return
print(f'Employee {name} not found')
def print_employee(name):
for emp in employees:
if emp['name'] == name:
print(f'Name: {emp["name"]}, Salary: {emp["salary"]}')
return
print(f'Employee {name} not found')
# Using functions
add_employee('Alice', 50000)
update_salary('Alice', 55000)
print_employee('Alice')
Here are a couple of problems with this approach.
- We have to pass the
name
around in every function to find the right employee. - If the data structure changes (e.g., adding a new field), we have to update multiple functions.
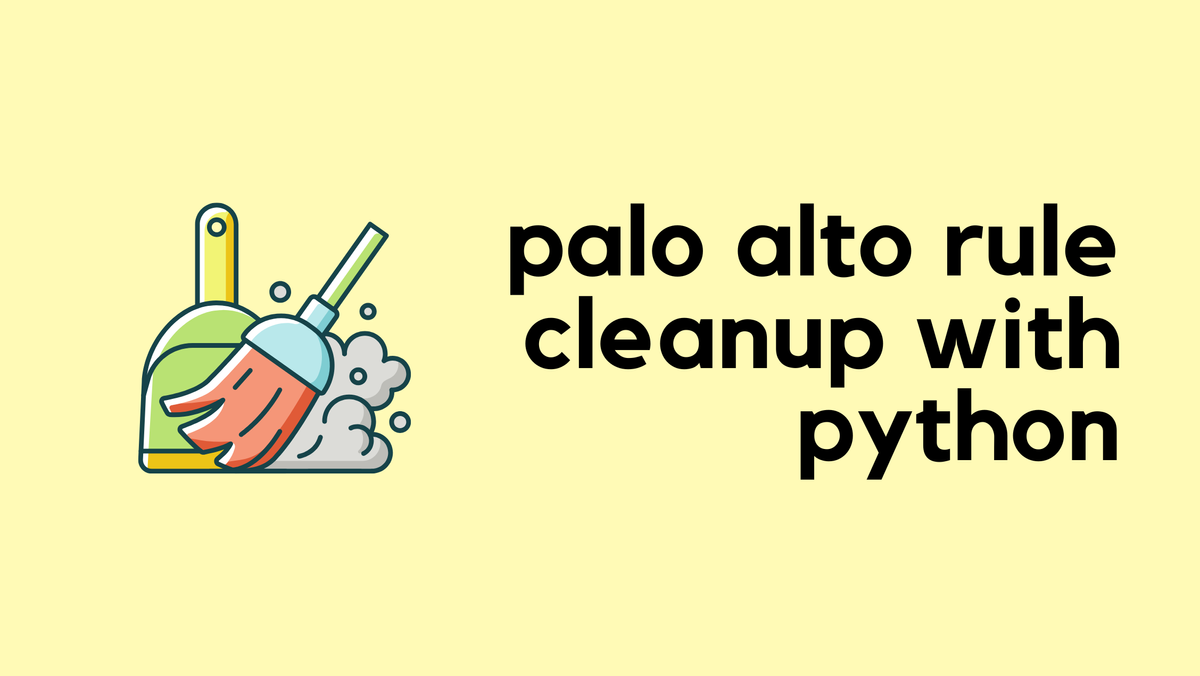
Managing Employees with a Class
An object in Python is an instance of a class. An object has attributes, which store data, and methods, which define behaviour. Think of a car, it has attributes like make, model, and colour, and it also has behaviours like accelerating or braking. Similarly, an employee object has attributes like name and salary and methods that allow updating or retrieving this information.
Using classes, we can organize our code in a way that makes it easier to manage and scale. Now, let’s see how we can improve this using a class.
class Employee:
def __init__(self, name, salary):
self.name = name
self.salary = salary
def update_salary(self, new_salary):
self.salary = new_salary
def print_details(self):
print(f'Name: {self.name}, Salary: {self.salary}')
alice = Employee('Alice', 50000)
alice.update_salary(55000)
alice.print_details()
The __init__
method is a special method that runs when a new object is created. It initializes attributes like name
and salary
. The self
parameter refers to the instance of the class, allowing access to its attributes and methods. When we create alice = Employee('Alice', 50000)
, it creates a new Employee
object with the given name and salary.
When we create an object from a class, we are creating an instance of that class. An instance is a unique object that has its own separate data. The class acts as a blueprint, and each instance is a distinct entity based on that blueprint. For example, alice = Employee('Alice', 50000)
creates an Employee
instance with its own name and salary attributes. If we create another instance, it will have its own independent values, separate from Alice’s data.
Instead of managing multiple dictionaries or lists, each Employee
object keeps track of its own details. This structure avoids the need for global variables and keeps the related data and functions together in a single place.
Expanding the Employee Class
Now that we have a basic class, let’s extend it by adding more functionality. We’ll add a department field and a method to give a raise.
class Employee:
def __init__(self, name, salary, department):
self.name = name
self.salary = salary
self.department = department
def update_salary(self, new_salary):
self.salary = new_salary
def give_raise(self, percentage):
self.salary += self.salary * (percentage / 100)
def print_details(self):
print(f'Name: {self.name}, Salary: {self.salary}, Department: {self.department}')
alice = Employee('Alice', 50000, 'Engineering')
bob = Employee('Bob', 60000, 'HR')
alice.give_raise(10)
alice.print_details()
bob.print_details()
We added a department
attribute to categorize employees and introduced a give_raise
method to update salaries based on a percentage. Now, managing employee data is even easier and more structured.
Conclusion
If your Python scripts are starting to get complex and hard to manage, classes can help you keep things organized. In this example, we saw how moving from functions to a class-based approach made managing employees easier and more intuitive. Classes let you group related data and behavior together, making your code cleaner and easier to work with. Once you get comfortable with this, you can start exploring more advanced topics like inheritance and polymorphism, but for now, this is a solid starting point.