If you're a Network Engineer curious about Terraform but not quite sure why you need it or how it can help, this blog post is just for you. I'm going to keep it straightforward and break down what Terraform and Infrastructure as Code (IaC) are all about, and importantly, why they're worth your time as a network engineer.
What is Terraform or IaC?
Terraform is a powerful tool that enables the management and provisioning of infrastructure through code. This is part of a broader practice known as Infrastructure as Code (IaC). With IaC, you handle all your infrastructure – whether it's network components, servers, or cloud environments – much like you would manage software. You write code to define everything, which allows for automation, consistency, and easier replication or adjustments.
Understanding Terraform becomes easier when we consider a practical scenario. Let's take an example from AWS. Suppose you need to create a VPC and then a subnet within that VPC. Traditionally, you'd navigate to the AWS VPC Service, manually create a new VPC, and then set up a subnet inside it. This process, especially when repeated across multiple accounts, can be tedious and time-consuming.
However, with Terraform, you can achieve the same result through code, here’s a glimpse of what this looks like in Terraform.
resource "aws_vpc" "test-vpc" {
cidr_block = "10.200.0.0/16"
tags = {
Name = "suresh-test"
}
}
resource "aws_subnet" "test-public-subnet" {
vpc_id = aws_vpc.test-vpc.id
cidr_block = "10.200.200.0/24"
map_public_ip_on_launch = "true"
availability_zone = "eu-west-1a"
tags = {
Name = "suresh-public-subnet"
}
}
This code snippet defines a VPC and a subnet. If you need to replicate this setup across multiple accounts or regions, it's as simple as adjusting a few lines in the code, rather than going through numerous GUI steps each time. This simplicity and scalability are what make Terraform an invaluable tool in modern infrastructure management.
Terraform for Netowork Automation
In the realm of Network Automation, Terraform stands out for its wide-ranging support across various network vendors. Big names like Cisco, Palo Alto, and Fortinet, among others, now integrate seamlessly with Terraform.
Take Palo Alto firewalls as an example. Traditionally, configuring a firewall involves navigating through its GUI, setting up rules, and making configuration changes – a process that needs to be repeated for each firewall. With Terraform, you define all these configurations in code. When you need to configure another firewall, it's just a matter of making minor adjustments in your Terraform code and applying it. This not only saves time but also ensures consistency across your firewall configurations.
Another key advantage of Terraform is its 'state' management. Unlike tools like Ansible, which are great for automation but don't keep track of the state of your resources, Terraform maintains a state file. This file records the current state of your resources as defined by your most recent Terraform apply
. The benefit here is significant, it allows Terraform to understand what has changed since the last run, enabling precise updates without needing to re-apply the entire configuration. This state-based approach provides a clear and accurate view of your infrastructure, making management and scaling much more efficient.
Back to AWS or Cloud
While Terraform's capabilities in Network Automation are impressive, I find its use with cloud providers like AWS to be particularly very useful.
Anyone who's worked with AWS can relate to the time and effort it takes to set up infrastructure components like VPCs, route tables, EC2 instances, and security groups through the GUI. It's not just the setup, the teardown is equally time-consuming, as you have to remove each component one by one.
Terraform simplifies this entire process. You deploy everything with a single command and, when you're done, you destroy it all with another. It's that straightforward.
For example, I have a Terraform file to deploy a Linux instance. Whenever I need a server for testing, I just run this code, and in a few seconds, the server is up and running. Once I'm done, another command and everything is cleanly removed. No more tedious navigation through multiple GUIs, no more manual tear-downs. If you want to learn more about Terraform, please check out my other blog posts below.
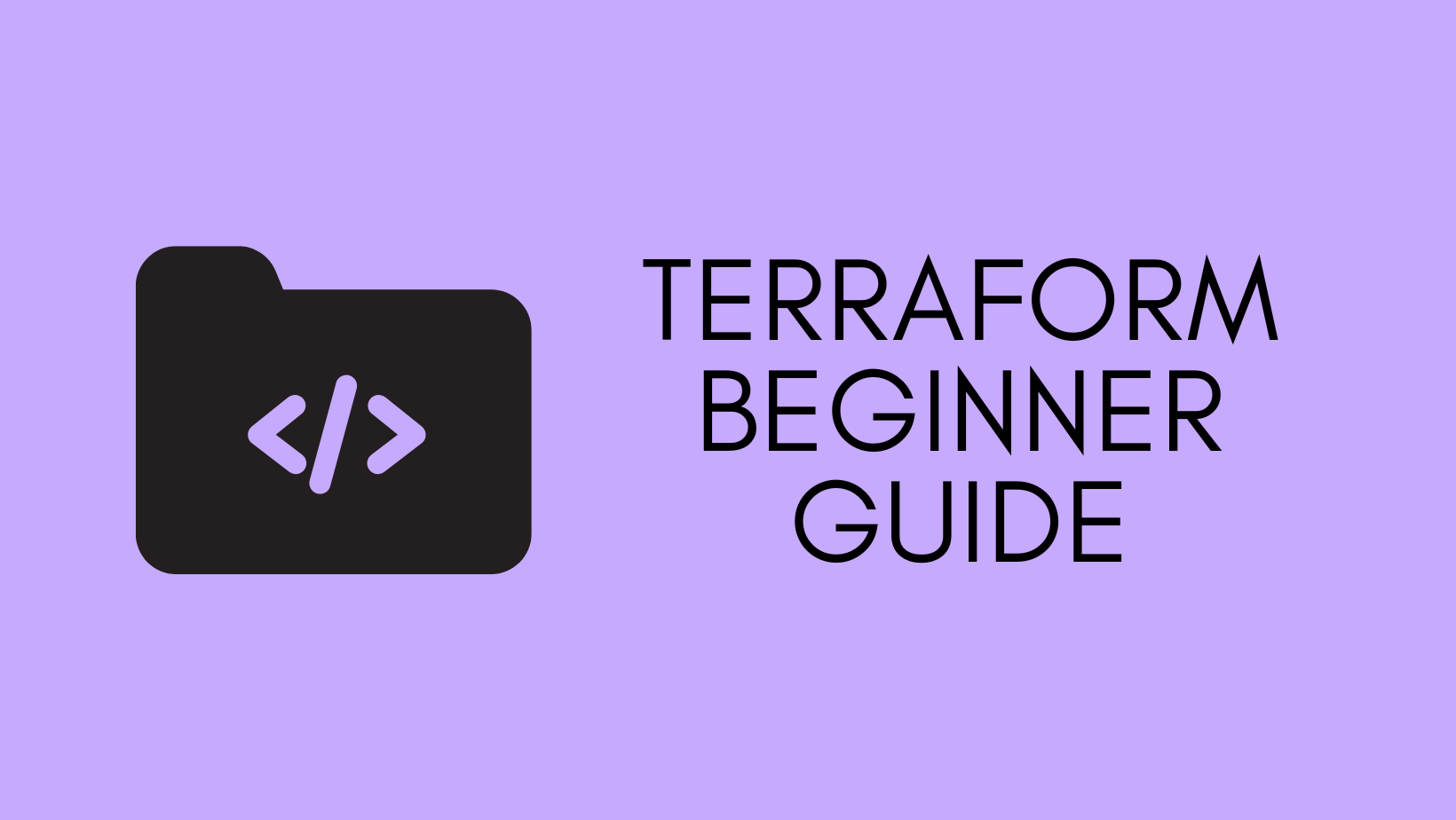
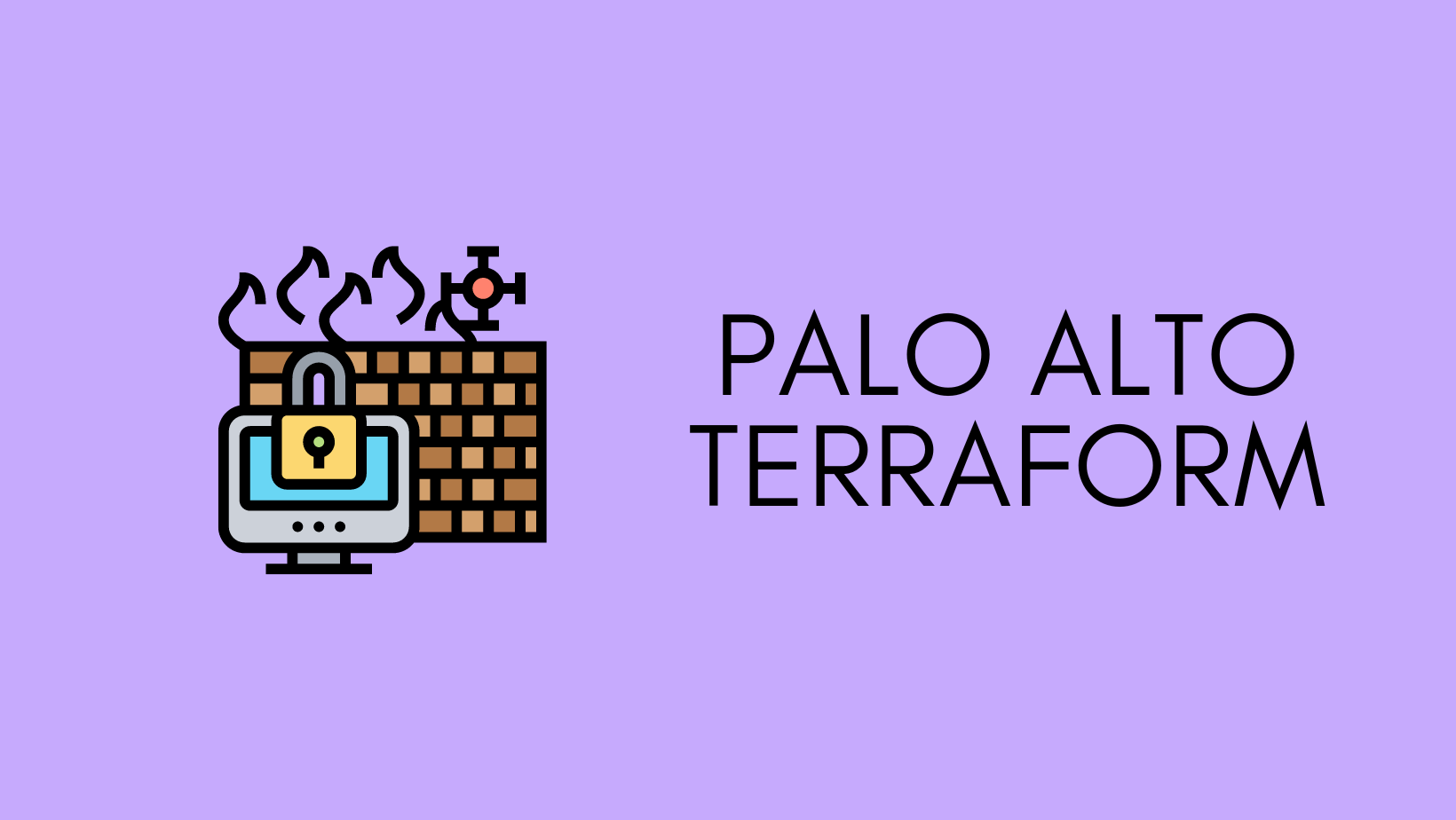
Learning Terraform is surprisingly easy. You might think that a tool this powerful requires months of learning, but that's not the case. In fact, you can get a good grasp of the basics in just a couple of days. The syntax is straightforward, and there's plenty of documentation and community support to help you along the way. Terraform is becoming more prevalent in the industry, and knowing Terraform can really boost your professional toolkit.
Example - Creating AWS Resources
As I mentioned before, one common task I frequently perform is spinning up Linux instances on AWS for testing. This requires not just the instance itself, but also setting up subnets, security groups, and other necessary resources. Here’s a Terraform file that I regularly use for this purpose. It's a straightforward yet powerful way to quickly get an EC2 instance up and running. Once my testing is done, a simple terraform destroy
command cleans up everything.
resource "aws_vpc" "test-vpc" {
cidr_block = "10.200.0.0/16"
tags = {
Name = "suresh-test"
}
}
resource "aws_subnet" "test-public-subnet" {
vpc_id = aws_vpc.test-vpc.id
cidr_block = "10.200.200.0/24"
map_public_ip_on_launch = "true"
availability_zone = "eu-west-1a"
tags = {
Name = "suresh-public-subnet"
}
}
resource "aws_route_table" "public-route" {
vpc_id = aws_vpc.test-vpc.id
tags = {
Name = "suresh-public-route"
}
}
resource "aws_internet_gateway" "test-igw" {
vpc_id = aws_vpc.test-vpc.id
tags = {
Name = "suresh-test-igw"
}
}
resource "aws_route" "internet-access" {
route_table_id = aws_route_table.public-route.id
destination_cidr_block = "0.0.0.0/0"
gateway_id = aws_internet_gateway.test-igw.id
}
resource "aws_route_table_association" "subnet-association" {
subnet_id = aws_subnet.test-public-subnet.id
route_table_id = aws_route_table.public-route.id
}
resource "aws_security_group" "allow_ssh" {
name = "allow_ssh_suresh"
description = "Allow SSH inbound traffic from my IP"
vpc_id = aws_vpc.test-vpc.id
ingress {
description = "SSH from GP"
from_port = 22
to_port = 22
protocol = "tcp"
cidr_blocks = ["1.1.1.1/32"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
ipv6_cidr_blocks = ["::/0"]
}
tags = {
Name = "suresh-test"
}
}
resource "aws_instance" "test-linux" {
ami = "ami-07355fe79b493752d"
instance_type = "t2.micro"
subnet_id = aws_subnet.test-public-subnet.id
key_name = "suresh-keypair"
vpc_security_group_ids = [ aws_security_group.allow_ssh.id ]
associate_public_ip_address = true
tags = {
Name = "suresh-test-linux"
}
}
output "public_ip" {
value = aws_instance.test-linux.public_ip
}
Let's break down what this code does at a high level.
- VPC and Subnet Creation - It starts by defining a VPC and a subnet within that VPC.
- Route Table and Internet Gateway - Next, it sets up a route table and an internet gateway.
- Security Group - It includes a security group configuration, which is crucial for defining the ingress and egress rules, like allowing SSH access.
- EC2 Instance - Finally, it spins up an EC2 instance in the defined subnet, with all the necessary configurations like security group, public IP association, etc.
By running this, all these components are provisioned in a coordinated manner, ensuring that the EC2 instance is ready for use within a minute. And when the resources are no longer needed, the terraform destroy
command ensures the clean removal of all these resources.
Final Thoughts
Just one more thought, learning Terraform and IaC is not just about technical efficiency, it's also a step towards future-proofing your skills. The industry is rapidly moving towards automation and cloud-based solutions. By learning Terraform, you're not only making your current tasks easier but also positioning yourself at the forefront of this evolving landscape.
This knowledge can open doors to new opportunities and collaborations, keeping you relevant and in-demand in the dynamic world of IT and network engineering. Remember, the investment you make in learning Terraform today will pay dividends in your career for years to come.